Introduction
Board games are a great way to have fun with friends and family! With the help of programming technology, you can create a digital version of your favorite board game in C++. In this tutorial, we will review the basics of setting up a board game in C++ and discuss best practices for improving coding efficiency.
Before beginning your project, you must first take time to familiarize yourself with the rules and setup of the board game. This includes learning about the number of players that can play at once, what pieces are used and how each turn should be played out. Taking notes as you learn is important for referencing behavior patterns when coding the structure of your game later on.
Once you have gathered all necessary information about the board game, deciding on a data structure for storing it is key. This can include using an array to keep track of every piece placed on the board or creating an object hierarchy to handle more complex games with more players and pieces. Structuring this data correctly is essential as it allows easier access when retrieving values like positions or next turns taken by players.
Once the data has been organized, it’s time to start coding the logic behind placing pieces on the gameboard! To do this, utilize core gameplay functions such as move(), attack(), or even setup(). These functions allow players not only make moves but also view available pieces and locations they may be moved to during their turn. Furthermore, these functions allow for more flexibility when mapping out complex strategies between multiple players in cooperative games.
By following these steps for setting up your board game using C++ programming language you’ll soon have an operational title ready for play! Have fun crafting your own unique gaming experiences by playing around with different source codes that suit your project best ” good luck!
Gather Necessary Materials
Building the Board – Utilizing a two-dimensional array, create the board using C++ code. Specify how many columns and rows should be included in the shape of the playing field.
Adding Images To The Board – Working on each piece separately, define images to include with the game board by utilizing external graphic libraries or rendering functions specific to C++.
Creating Pieces – Establish pathways and routes for the pieces depending on what type of game is being made. These can also be changed from one side of the square to another.
Assigning Numbers To Players – Assign different numbers to each player so that when it is their turn, their number can be easily identified and votes counted accordingly.
Placing Pieces On The Board And Capturing Opposing Pieces – Using numerical keys such as integers, assign players an action so that they can place pieces on any square they desire and capture opposing pieces if needed. Also determine how fast a turn should have to be made in order for a player’s move to be valid, if there are any time limits for making turns or specific rules related to moves on the board during gameplay.
Initiation Of Play Through Multiple Platforms – Create methods which allow multiple platforms within the game such as allowing users to play against one another online or through local networks or create audio soundtracks which can add an extra element of excitement when playing against others or provide background music while playing solo-player mode games.
Understand the Language
To write a program to set up a game board in C++, you will need to understand some of the fundamentals of the language. Primarily, this involves having a good grasp on how variables work – how they can store data and how they can be manipulated using loops, operators and functions. Additionally, you should understand object-oriented programming principles such as classes, objects and public/private access modes; these concepts allow you to better manage the code used to create complicated structures such as a game board.
When writing out your code, it is important to use consistent formatting and structure so that other people who may use your code later can easily follow along. You may also want to consider adopting best practices like creating meaningful variable names and utilizing comments throughout your code for easier readability.
Once you have an understanding of the key concepts needed for working with C++ and structuring your code appropriately, it’s time to start coding! Depending on the particular game that you are setting up a board for, there may be some specific requirements or constraints that will drive what type of data structures are necessary (such as arrays or linked lists) and the overall structure of the program. The most basic approach is to create a 2-dimensional array representing the game board itself, which each element then populated according to whatever rules define individual pieces in the game.
Designing the Board
When creating a player in C++ for a board game, it is important to first design the board. First, decide on the size and dimensions of your board and create it either with characters (e.g. ‘-‘ or ‘o’) or with graphical representations of the pieces used on the board game. Generally, a two-dimensional array or string can be used to represent the rows and columns of a board’s design. For example, if the size of the board was 8×8 then an 8 element strings array could be created for each row:
string sampleBoard[8];
Once you have designed the general shape of your board game, you must decide what pieces will populate it. This could include anything from tokens to dice and character pieces. These can be represented by either numbers (e.g 1-6) or symbols that have assigned values depending on the rules of the game. For example, you may choose to use ‘P’ as a player token:
sampleBoard[2][4] = ‘P’;
Apart from deciding on what tokens populate your board map you must also consider other user settings such as movement restrictions or victory conditions whenever applicable. When determining these rules one must also take into consideration how players interact not only with each other but also their environment (i.e., are they allowed to move off-grid?). There are many online resources available details specific strategies for designing boards games in C++ which should act as a useful starting point for any developer hoping to create their own virtual interactive experience using this programming language.
Program Your Turn
1. Create a list of possible moves a player can make and store them in an array. Depending on the type of game, this may include moves like rolling dice, moving pieces around the board, or using cards to influence events.
2. Determine how each action will affect the board’s context. For example, if a player rolls a die to move forward, then record that number and adjust the location of their piece accordingly. Similarly, if they draw a card from a deck, note what effect it has on the game state such as adding or subtracting resources or changing rules for future turns.
3. Set any conditions for making certain moves such as requiring that players be within specific boundaries or cannot move onto spaces occupied by other players and objects. Add these conditions as part of your program’s logic so that it handles and validates user input correctly.
4. Designate start and end locations so that when players reach either one in an action sequence (dice roll), they are either sent back to the beginning or progress to the next turn accordingly.
5. Calculate scores by keeping track of data like points earned based on completing tasks or going around game boards multiple times before it ends in “victory” condition usually defined at start (e.g., last person with most points wins).
6. Debug your code before introducing it into the game by testing various scenarios to ensure that all possibilities have been considered and programmed for accurately.
Advanced Features and Options
When setting a player on a board game written in C++, it is important to consider any advanced features or options. These features can include choices of different players, in-game items and abilities, turn limits, and other variables that may fluctuate throughout the game. Depending on what kind of board game you are creating, these components can be added and modified to suit the player’s needs. For instance, if the board game requires two players with distinct roles, each character could be assigned specific abilities or items. Scoreboards or a timer also may be added to account for when a player’s turn should end and points should be tracked. After adding all such additional possibilities and parameters into the code, you work towards providing an enjoyable gaming experience that fits with ‘the theme’ of the board game being produced.
Testing
1. Begin by writing down a list of the game’s current rules. Review the list and make sure that you understand how every rule applies in your game.
2. Create a program or script to test each rule as you set up different game scenarios. This will help to ensure all rules are followed during set up and gameplay.
3. If using a program, start by setting up the environment for the player to be placed in the game. If hand-coding, define variables related to the players’ attributes and store them in memory (e.g., name, score, etc.).
4. Assign each player a unique identifier that won’t be confused with any other player’s ID during setup (e.g., label each player A – Z for board games).
5. Write code to check whether or not all players have their turn in the game before continuing on with other parts of the program (i.e., verify no one is skipped).
6. Depending on your game, have code run checks against all players’ statuses such as remaining health points or number of pieces they still have available on the boardgame at any given time before allowing moves forward in your program flow (if applicable/needed).
7. Ensure that all data related to each player including scores, inventory tracking if applicable) is being updated correctly after every move throughout gameplay in accordance with defined rules and regulations within C++ language itself.
Debugging – Step-by-step instructions on how to debug these tests:
8 . Debugging should always begin by verifying that all values used throughout testing (specifically those related to individual players) are declared correctly according their expected types established priorly within C++ language designations themselves (i.e., strings for names or numbers for scores).
9 . Verify also that necessary variables are initialized properly before use as these can cause certain discrepancies when reading memory as well from invalid declared statements entailing different keywords associated with individual attribute tracking systems throughout gameplay itself versus those contained solely within custom coding specifics .
10 . Pay special attention out for undefined parameters regarding character movement within the board gaming logic itself as not accounting these while debugging can potentially throw unexpected results when comparing values between two phases of testing procedures following any changes which may have recently been made here alone afterwards too upon resolving issues otherwise noticed differently elsewhere beforehand at earlier stages even then lastly too as well overall still though instead
Conclusion
Setting up a board game in C++ is not a difficult process. It requires basic knowledge of the language and understanding of how it functions.
Using a two-dimensional array, each location on the board can be identified and stored as an element in this array. Furthermore, users should define functions to move players around the board, such as those that accept input from players and those that update the elements within the array. Players’ turns can also be manipulated using control structures like loops or switches, allowing for greater control of the game flow. Once these basic mechanisms are established, game logic rules can be applied to define how a particular turn should proceed (e.g what happens when one player lands on another’s property).
In order to set up a board game in C++, users must understand data structures and algorithms well enough to compile them into an executable program. Furthermore, sufficient debugging is necessary to ensure that all code is working correctly. With careful planning and attention to detail, developers should find setting up a board game in C++ manageable and enjoyable task.
Overall, setting up a board game in C++ involves defining locations on the board with a two-dimensional array, using various functions and control structures to initiate turns by players correctly, applying appropriate game logic rules to determine what should happen during certain turns such as when one player lands on another’s property; and ensuring accurate compilation and debugging processes when building the program executable.
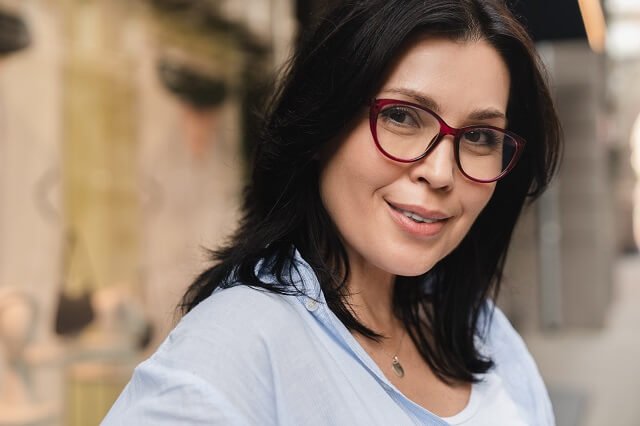
I love playing all kinds of games – from classics like Monopoly to modern favourites like Ticket to Ride.
I created this blog as a way to share my love of board games with others, and provide information on the latest releases and news in the industry.